AI prompts
base on QuestPDF is a modern open-source .NET library for PDF document generation. Offering comprehensive layout engine powered by concise and discoverable C# Fluent API. Easily generate PDF reports, invoices, exports, etc. <a href="https://www.questpdf.com/" target="_blank">
<img src="https://github.com/QuestPDF/example-invoice/raw/main/images/logo.svg" width="400">
</a>
---
[](https://www.questpdf.com)
[](https://www.nuget.org/packages/QuestPDF/)
[](https://github.com/QuestPDF/QuestPDF/stargazers)
[](https://www.nuget.org/packages/QuestPDF/)
[](https://www.nuget.org/packages/QuestPDF/)
[](https://www.questpdf.com/license/)
<br />
### QuestPDF is a modern open-source .NET library for PDF document generation. Offering comprehensive layout engine powered by concise and discoverable C# Fluent API.
```csharp
Document
.Create(document =>
{
document.Page(page =>
{
page.Size(PageSizes.Postcard);
page.Margin(0.3f, Unit.Inch);
page.Header()
.Text("Hello PDF!")
.FontSize(28)
.Bold()
.FontColor(Colors.Blue.Darken2);
page.Content()
.PaddingVertical(8)
.Column(column =>
{
column.Spacing(8);
column.Item()
.Text(Placeholders.LoremIpsum())
.Justify();
column.Item()
.AspectRatio(16 / 9f)
.Image(Placeholders.Image);
});
page.Footer()
.AlignCenter()
.Text(text =>
{
text.Span("Page ");
text.CurrentPageNumber();
});
});
})
.GeneratePdfAndShow();
```
<img src="https://github.com/user-attachments/assets/ceb5fbbb-843e-46ae-97c1-082a704e8a99" width="300">
<br />
<br />
<br />
## Please help by giving a star
GitHub stars guide developers toward great tools. If you find this project valuable, please give it a star – it helps the community and takes just a second! ⭐
<img src="https://github.com/user-attachments/assets/bbc7cdc6-ac09-4bb3-bb9d-8abfa81e79fb" width="700" />
<br />
## QuestPDF Companion App
Accelerate your development with live document preview powered by the hot-reload capability, eliminating the need for code recompilation:
- Explore document structure and hierarchy
- Quickly magnify and measure content
- Debug runtime exceptions with stack traces and code snippets
- Identify, understand and solve layout errors
<picture>
<source media="(prefers-color-scheme: dark)" srcset="https://github.com/user-attachments/assets/7ab596d4-eebc-44e6-b36d-c358b16ed0ba">
<source media="(prefers-color-scheme: light)" srcset="https://github.com/user-attachments/assets/39d4c08c-6a78-4743-8837-208c0c1718fd">
<img src="https://github.com/user-attachments/assets/ce394258-1f10-498d-b65f-26c9fbed2994" width="600">
</picture>
[](https://www.questpdf.com/companion/features.html)
<br />
## What you need is here
`Comprehensive Layout Engine` - A layout engine tailored for document generation, offering advanced paging and precise content control.
`Rich Toolkit` - Craft documents with intuitive, reusable components and over 50 layout elements for complex designs.
`High Performance` - Generate thousands of pages per second with minimal CPU and memory usage.
`Advanced Language Support` - Seamlessly create multilingual documents with support for RTL, text shaping, and bi-directional content.
<br />
## Code-Focused Paradigm
Using C# to design PDF documents leverages powerful control structures like if-statements, for-loops, and methods, enabling dynamic and highly customizable content generation.
It promotes best practices such as modular design and reusability while seamlessly integrating with source control systems for collaboration and versioning.
```csharp
.Column(column =>
{
if (Model.Comments != null)
column.Item().Text(Model.Comments);
foreach(var item in Model.Items)
column.Item().Element(c => CreateItem(c, item);
});
```
```diff
void CreateItem(IContainer container, Item item)
{
container
- .Background(Colors.Grey.Lighten2)
+ .Background(item.Color)
.Padding(10)
.Text(item.Text);
}
```
<br />
## Multiplatform
The library supports all major operating systems, integrates seamlessly with leading IDEs as well as popular cloud platforms and technologies to ensure maximum flexibility.
- `Technologies`: modern dotnet, legacy .NET Framework, Docker
- `Operating systems`: Windows, Linux, MacOS
- `Cloud providers`: Azure, AWS, Google Cloud
- `IDE`: Visual Studio, Visual Code, JetBrains Rider, others
<br />
## Perform common PDF operations
- Merge documents
- Attach files
- Extract pages
- Encrypt / decrypt
- Extend metadata
- Limit access
- Optimize for Web
- Overlay / underlay
```csharp
DocumentOperation
.LoadFile("input.pdf")
.TakePages("1-10")
.MergeFile("appendix.pdf", "1-z") // all pages
.AddAttachment(new DocumentAttachment
{
FilePath = "metadata.xml"
})
.Encrypt(new Encryption256Bit
{
OwnerPassword = "mypassword",
AllowPrinting = true,
AllowContentExtraction = false
})
.Save("final-document.pdf");
```
[](https://www.questpdf.com/concepts/document-operations.html)
<br />
## Let's get started
Follow our detailed tutorial, and see how easy it is to produce a fully functional invoice with fewer than 250 lines of C# code.
[](https://www.questpdf.com/getting-started.html)
<img src="https://github.com/QuestPDF/QuestPDF-Documentation/blob/main/docs/public/invoice-small.png?raw=true" width="400px">
<br />
## Sustainable and Fair License
By offering free access to most users and premium licenses for larger organizations, the project maintains its commitment to excellence while ensuring sustainable, long-term development for all.
> [!WARNING]
> The library is free to use for any individual or business with less than 1 million USD annual gross revenue, or operates as a non-profit organization, or is a FOSS project.
[](https://www.questpdf.com/license/)
<br />
## QuestPDF on YouTube
We are incredibly grateful to the YouTube Community for their positive reviews and recommendations of the QuestPDF library. Your support and feedback are invaluable and motivate us to keep improving and expanding this project. Thank you for helping us grow and reach more developers!
#### Nick Chapsas: The Easiest Way to Create PDFs in .NET
[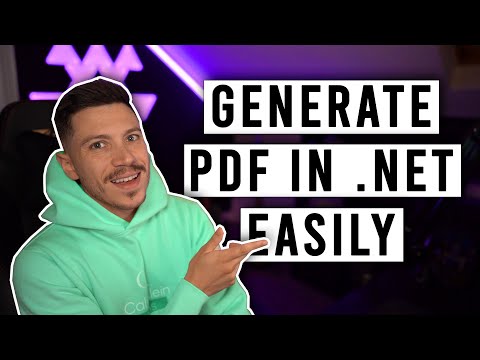](https://www.youtube.com/watch?v=_M0IgtGWnvE)
#### Claudio Bernasconi: QuestPDF - The BEST PDF Generator for .NET?!
[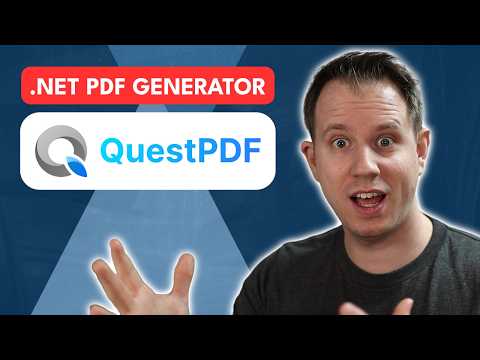](https://www.youtube.com/watch?v=T89A_7dz1P8)
#### JetBrains: OSS Power-Ups: QuestPDF
[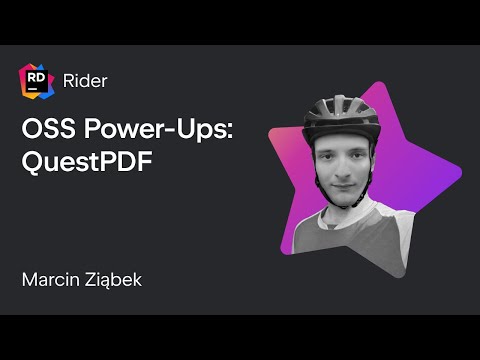](https://www.youtube.com/watch?v=-iYvZvpLX0g)
#### Programming with Felipe Gavilan: Generating PDFs with C# - Very Easy (two examples)
[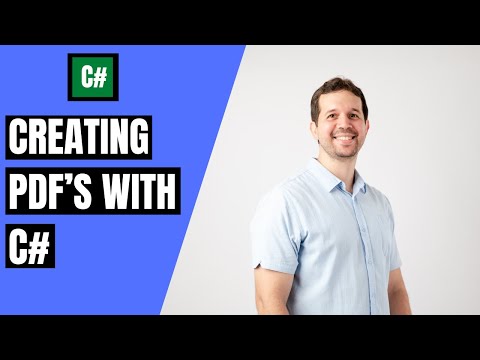](https://www.youtube.com/watch?v=bhR4Cmg16gs)
", Assign "at most 3 tags" to the expected json: {"id":"3017","tags":[]} "only from the tags list I provide: [{"id":77,"name":"3d"},{"id":89,"name":"agent"},{"id":17,"name":"ai"},{"id":54,"name":"algorithm"},{"id":24,"name":"api"},{"id":44,"name":"authentication"},{"id":3,"name":"aws"},{"id":27,"name":"backend"},{"id":60,"name":"benchmark"},{"id":72,"name":"best-practices"},{"id":39,"name":"bitcoin"},{"id":37,"name":"blockchain"},{"id":1,"name":"blog"},{"id":45,"name":"bundler"},{"id":58,"name":"cache"},{"id":21,"name":"chat"},{"id":49,"name":"cicd"},{"id":4,"name":"cli"},{"id":64,"name":"cloud-native"},{"id":48,"name":"cms"},{"id":61,"name":"compiler"},{"id":68,"name":"containerization"},{"id":92,"name":"crm"},{"id":34,"name":"data"},{"id":47,"name":"database"},{"id":8,"name":"declarative-gui "},{"id":9,"name":"deploy-tool"},{"id":53,"name":"desktop-app"},{"id":6,"name":"dev-exp-lib"},{"id":59,"name":"dev-tool"},{"id":13,"name":"ecommerce"},{"id":26,"name":"editor"},{"id":66,"name":"emulator"},{"id":62,"name":"filesystem"},{"id":80,"name":"finance"},{"id":15,"name":"firmware"},{"id":73,"name":"for-fun"},{"id":2,"name":"framework"},{"id":11,"name":"frontend"},{"id":22,"name":"game"},{"id":81,"name":"game-engine "},{"id":23,"name":"graphql"},{"id":84,"name":"gui"},{"id":91,"name":"http"},{"id":5,"name":"http-client"},{"id":51,"name":"iac"},{"id":30,"name":"ide"},{"id":78,"name":"iot"},{"id":40,"name":"json"},{"id":83,"name":"julian"},{"id":38,"name":"k8s"},{"id":31,"name":"language"},{"id":10,"name":"learning-resource"},{"id":33,"name":"lib"},{"id":41,"name":"linter"},{"id":28,"name":"lms"},{"id":16,"name":"logging"},{"id":76,"name":"low-code"},{"id":90,"name":"message-queue"},{"id":42,"name":"mobile-app"},{"id":18,"name":"monitoring"},{"id":36,"name":"networking"},{"id":7,"name":"node-version"},{"id":55,"name":"nosql"},{"id":57,"name":"observability"},{"id":46,"name":"orm"},{"id":52,"name":"os"},{"id":14,"name":"parser"},{"id":74,"name":"react"},{"id":82,"name":"real-time"},{"id":56,"name":"robot"},{"id":65,"name":"runtime"},{"id":32,"name":"sdk"},{"id":71,"name":"search"},{"id":63,"name":"secrets"},{"id":25,"name":"security"},{"id":85,"name":"server"},{"id":86,"name":"serverless"},{"id":70,"name":"storage"},{"id":75,"name":"system-design"},{"id":79,"name":"terminal"},{"id":29,"name":"testing"},{"id":12,"name":"ui"},{"id":50,"name":"ux"},{"id":88,"name":"video"},{"id":20,"name":"web-app"},{"id":35,"name":"web-server"},{"id":43,"name":"webassembly"},{"id":69,"name":"workflow"},{"id":87,"name":"yaml"}]" returns me the "expected json"